Detailed instructions for the Devolutions Server REST API are available in the Apps and companion tools section within the Devolutions Server web interface in Help & Tools – API documentation.
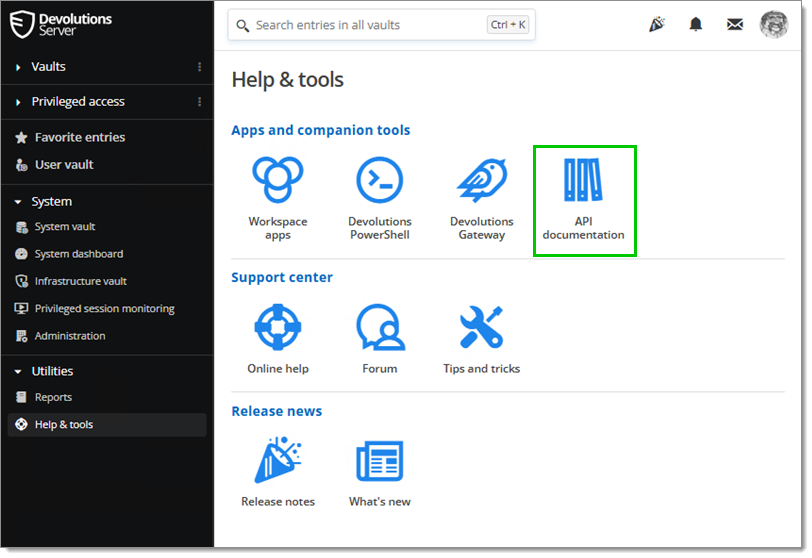
Here is what the REST API window looks like:
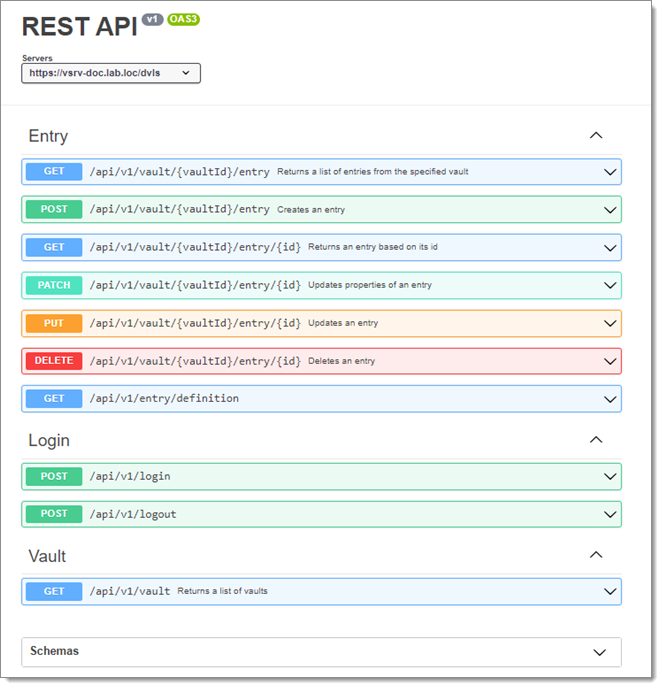
To connect and use the REST API, refer to the sample bash script below. For each API call to the server, ensure the following:
Replace
https://url
with the specific Devolutions Server URL.Replace
GUID-OF-VAULT
andGUID-OF-ENTRY
with the corresponding GUIDs.
In this version of the API, passwords can only be retrieved from entries of the Credentials type.
# Log in and get the token
authResponse=$(curl -s -X POST "https://url/api/v1/login" \
-H "Content-Type: application/json" \
-d '{
"appKey": "appkey",
"appSecret": "appsecret"
}')
token=$(echo $authResponse | jq -r '.tokenId')
# Check if the token was successfully obtained
if [ -z "$token" ] || [ "$token" = "null" ]; then
echo "Failed to login or obtain token."
exit 1
fi
# Request data using the token
response=$(curl -s -X GET "https://url/api/v1/vault/GUID-OF-VAULT/entry/GUID-OF-ENTRY" \
-H "Content-Type: application/json" \
-H "tokenId: $token")
echo $response | jq
# Log out
curl -s -X POST "https://url/api/v1/logout" \
-H "Content-Type: application/json" \
-H "tokenId: $token" \
-H "Content-Length: 0"